CATIA macro selection
Introduction
CATIA macro selection is a very important topic to learn when automating processes. It is ground in every macro. Hence for every action in a macro, you need some kind of selection. There are single and multiple ones. There are also two ways to select elements. So those are user selection and search by name. Hence when you have the selection you want to use that for loop control. For example, if you select 4 bodies you can iterate 4 times. So in this post, we will show you more examples of selection usage.
Example 1: Change color
Comments are inserted in code. So you will follow and better understand it. Let’s start with a simple selection code so you understand it first.
'Every CATIA macro starts with Sub CATMain and ends with End Sub, in between is your macro code, except methods. Sub CATMain() 'First you need to declare new variable for selection. Dim oSel as Selection 'In next row you set the selection, and you can see it's ActiveDocument, it means that you can select everywhere. Set oSel=CATIA.ActiveDocument.selection 'Now we can use that selection and in this case we want to change color. oSel.VisProperties.SetRealColor 255, 0, 0, 0 End Sub 'This is just simple example of selection and usage of it. 'In next video you can see how can it be very useful.
Example 2: Single selection for new add
In example 2 we want to use CATIA selection for selecting the body. We want to add it to another body. For this, we will use a simple selection. So it means that a user will be available to select only one input. As a result, we should decide which selection we need for our code.
Language="VBSCRIPT" Sub CATMain() 'First you need to define document, in this case active document is part. Selection also will be in part. Dim partDocument1 As Document Set partDocument1 = CATIA.ActiveDocument Dim part1 As Part Set part1 = partDocument1.Part Dim oSel as Selection Set oSel = partDocument1.selection 'We want to set filter for that selection so user can only select part, this is very good practice to do Dim Filter(0) Filter(0)="Body" Dim F_Body as object 'In next line of code is code for single selection, also this code is always the same. You just need to remember or write it down F_Body=oSel.selectelement2(Filter, " Select Body in which you want to add", False) 'Because we work with bodies, we need to define them Dim bodies1 As Bodies Set bodies1 = part1.Bodies 'We will use that name from selection to define our first body Dim body1 As Body Set body1 = bodies1.Item(oSel.item(1).value.name) ' This first selected body is inworkobject, it means that macro will define in this body. Every time you need to define in body you will use this code. part1.InWorkObject = body1 'Before we want to select our second body we need to clear the selection. When selection is clear we can let user to make selection. oSel.clear F_Body=oSel.selectelement2(Filter, " Select Body which you want to add", False) Dim body2 As Body Set body2 = bodies1.Item(oSel.item(1).value.name) ' In the end we can add our second body in the first. We need some declaration for that add to. If you don't know how to declare it, you can always record macro or you can check in V5Automation.chm file.We will put file for download and picture of it. Dim shapeFactory1 As Factory Set shapeFactory1 = part1.ShapeFactory shapeFactory1.AddNewAdd body2 'After add you need to update this part, you can also update just feature. Updating only feature is much better option for big models because it takes less time. part1.Update 'You want to display message to inform user that macro is done. msgBox("DONE!!" & body2.name & " added into " & body1.name) End Sub
If you want to test this CATIA macro code you can just copy it. So you can try to use an input box to set and add a name for the body. You can watch my video tutorial to understand better.
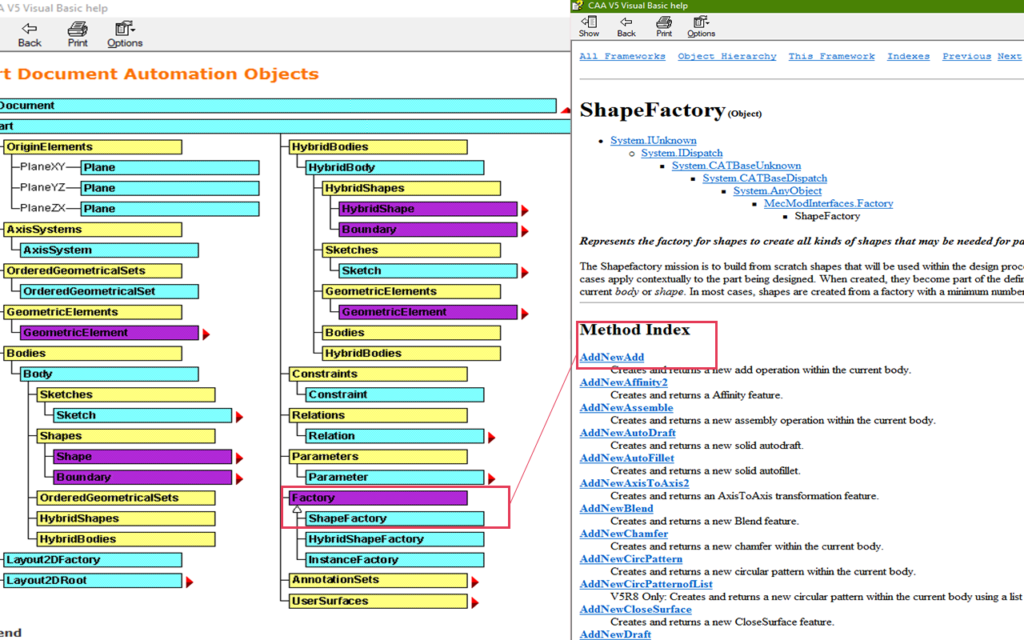
This is a preview of the automation file. This file can help you a lot. So in this file, you can find everything you need.
Example 3:Multiple selection for new add
This macro is very similar to the previous one. In contrast in this, we want to use multiple selection.
Language="VBSCRIPT" Sub CATMain() Dim partDocument1 As Document Set partDocument1 = CATIA.ActiveDocument Dim part1 As Part Set part1 = partDocument1.Part Dim oSel as Selection Set oSel = partDocument1.selection Dim Filter(0) Filter(0)="Body" Dim F_Body as object F_Body=oSel.selectelement2(Filter, " Select Body in whic you want to add", False) Dim bodies1 As Bodies Set bodies1 = part1.Bodies Dim body1 As Body Set body1 = bodies1.Item(oSel.item(1).value.name) part1.InWorkObject = body1 oSel.clear 'This line of code is different, here we now want to select multiple bodies. This is code for multiple selection and you can use it for every multiple selection. When you run multiple selection you will get palette for selection. F_Body = oSel.SelectElement3(Filter, "Select Bodies whic you want to add", False, CATMultiSelectionMode.CATMultiSelTriggWhenUserValidatesSelection, False) Dim shapeFactory1 As Factory Set shapeFactory1 = part1.ShapeFactory 'We want to control this for loop with our multiple selection for i=1 to oSel.count Dim body2 As Body Set body2 = bodies1.Item(oSel.item(i).value.name) 'We can set the name for the body on this way and also we will set the name for the add on the same way body2.name="CATIA_MACROS__" & i ShapeFactory1.AddNewAdd (body2).name="ADD_CATIA_MACROS__" & i part1.Update next msgBox("DONE!!" & oSel.count & " added into " & body1.name) End Sub
Example 4: CATIA macro selection with search
Also in the next example, we want to show you how to make a selection with a search option. We will not comment on the same lines. Rather we will different ones.
Sub CATMain() Dim oSel as Selection Set oSel = CATIA.ActiveDocument.Selection Dim Name As String Name = Inputbox("Please enter name of geometry to search for.") 'In next line selection will search for name from inputbox inside all parts or products. oSel.Search "Name=" & Name & "*,all" 'When we made selection now we can use it iCount = oSel.Count msgbox "Number of shapes found: "&icount For i=1 to iCount CATIA.StartCommand "Center Graph" Next End Sub 'You can run this macro in part and product!!
This search option is very powerful when you define some basic names. Also, it’s very easy to connect with powercopy. If you want to read more about that go to this link.
You can also read more about selection on those links.
http://www.scripting4v5.com/additional-articles/catia-macro-selection/
http://www.scripting4v5.com/additional-articles/the-selection-object-and-search-function/
Hello,
First of all thank you for your website it is really interesting and very helpful.
I’m a student and a beginner in VBA Catia and I have a question: I’ll try and explain exactly my situation
i have a list of part numbers in excel : i succeed in connecting that with catia
now i want that for each cell of my range i call a macro. i have put all the cells in an array and i’m trying to code the following in a loop:
for each cell value:
1-look in the catia tree if it’s present
2- if it is call my macro: here the macro is to extract properties for each partnumber call walkdowntree (myProduct)
i don’t know how to link each array value to the myProduct to run the macro
3-if not msgbox: partnumber does not exist
Thank you in advance for any ideas or help
It will be appreciated
Hello Rih, thx for the nice words. In the beginning, I want to encourage you to go with VB, not with VBA. VB is much easier, more advanced and has many more options.
I didn’t figure out what is the main goal of your code? Do you want to get properties of those parts from the excel list? If you want that, then you can sent back those values in excel for each iteration??
Is this some sort of stocklist or something else?
Hello, Thank you for your answer
sorry i didn’t explain well what i need
well what i did for now is using the catia vb editor and i created a userform to ask the person for the list of the products that we need to extract the BOM for. what i managed to do is have a first button that will create a new excel file and ask for the listing.
then using another button it will launch a macro that will for each number listed extract the Bom and store it in an excel file. I couldn’t do the loop, i tried with putting only one iteration
and i couldn’t print the BOM
for the excel file i needed to be:
Product number | Reference | QTY
i don’t know if you can help me, i’m willing to send you what i did by email?
thanks again
-Rih
Hello, yes now it has more sense. How a person will make that list, by selection or? I can help you ofc if you want I can make VB app to do that. Also, I can check what did you do, and give you some suggestions. In both cases, it will take some time for me to do that so it will not be free, unfortunately :(. Harun
Hello,
Can you send me a message in private with the price
So we can discuss this
Is it achievable in 7days?
Hello, sent.